Creating WebVTT Files for Videos Using Node.js: A Comprehensive Guide
Darius Baruo Jun 18, 2024 07:16
Learn how to create WebVTT subtitle files for videos using Node.js and the AssemblyAI API in this detailed guide.
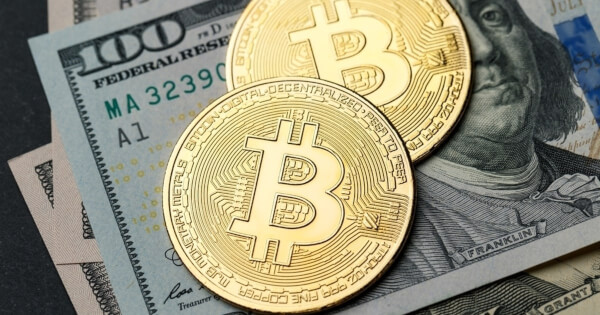
WebVTT (.vtt) or Web Video Text Tracks Format is a widely supported format for subtitles in videos. In this guide, you'll learn how to create WebVTT files for videos using Node.js and the AssemblyAI API, according to AssemblyAI.
Step 1: Set Up Your Development Environment
First, install Node.js 18 or higher on your system. Create a new project folder, change directories to it, and initialize a new Node.js project:
mkdir vtt-subtitles
cd vtt-subtitles
npm init -y
Open the package.json file and add type: "module",
to the list of properties:
{
...
"type": "module",
...
}
This will tell Node.js to use the ES Module syntax for exporting and importing modules. Then, install the AssemblyAI JavaScript SDK:
npm install --save assemblyai
Next, obtain an AssemblyAI API key from your dashboard. Set it as the ASSEMBLYAI_API_KEY
environment variable:
# Mac/Linux:
export ASSEMBLYAI_API_KEY=<YOUR_KEY>
# Windows:
set ASSEMBLYAI_API_KEY=<YOUR_KEY>
Step 2: Transcribe Your Video
With the development environment ready, start transcribing your video files. Use this sample video for practice. Create a file called index.js
and add the following code:
import { AssemblyAI } from 'assemblyai';
const client = new AssemblyAI({ apiKey: process.env.ASSEMBLYAI_API_KEY });
const transcript = await client.transcripts.transcribe({
audio: "https://storage.googleapis.com/aai-web-samples/aai-overview.mp4",
});
If the transcription is successful, the transcript
object will be populated. Verify and log any errors:
if (transcript.status === "error") {
throw new Error(transcript.error);
}
Step 3: Generate WebVTT File
Generate the subtitles in WebVTT format. Import the necessary module to save the WebVTT file to disk:
import { writeFile } from "fs/promises"
Add the following code to generate and download the VTT file:
const vtt = await client.transcripts.subtitles(transcript.id, "vtt");
await writeFile("./subtitles.vtt", vtt);
Customize the maximum number of characters per caption if needed:
const vtt = await client.transcripts.subtitles(transcript.id, "vtt", 32);
await writeFile("./subtitles.vtt", vtt);
Step 4: Run the Script
Run the script from your shell:
node index.js
After execution, you'll find a new file subtitles.vtt
on disk:
WEBVTT
00:00.200 --> 00:04.430
AssemblyAI is building AI systems to help you build AI applications with
00:04.462 --> 00:08.694
spoken data. We create superhuman AI models for speech recognition,
00:08.774 --> 00:13.062
summarization, knowledge, augmentation of large language models with spoken
Next Steps
Now that you have your subtitle file, you can configure it in your video player or upload it to YouTube Studio. Additionally, explore other tools to bundle or burn subtitles into your video. Check out AssemblyAI's Audio Intelligence models and LeMUR for more capabilities in your audio and video applications.
For more educational content, visit the AssemblyAI blog or their YouTube channel.
Image source: Shutterstock